In every programming environment a common use case is to handle a collection of data. In PowerApps the data type for collections are simply PowerApps Collections. This guide will show you how to create, clear, add and delete item with PowerApps Collections.
The article should function as reference for you and me. In case you find something that is wrong, could be achieved in a better way or you are simple missing something, just let me know within the comments or contact me.
What is a PowerApps Collection?
PowerApps collections is a data type to store a group of items in memory. PowerApps provides multiple functions to create, add, update, remove, filter and sort the items within a collection.
Before we get into detail on these functions, let’s get an idea of a collection by an example of a collection.
PowerApps Collection example
Why use collections? Let’s image you want to implement an address book. An address book is a collection of contact data. Within PowerApps you can group the contact data with PowerApps collections.
Your collection of contact data might look like this:
Name | Age | |
---|---|---|
John Doe | 45 | [email protected] |
Maria Smith | 23 | [email protected] |
Mike Miller | 33 | [email protected] |
Read how to create the collection within PowerApps.
Working with PowerApps Collections
How do I create a collection?
A new PowerApps collection is created with the Collect-Function. Below we create a collection for the example data. A collection with the name “Contacts” is created containing our three contacts (John, Maria and Mike).
An alternative is the ClearCollect-function. It does the same thing, but it does remove (clear) all items that might already be in the collection. The ClearCollct function is useful whenever you have some code snippet that is executed multiple times.
Collect(
Contacts,
{
Name: "John Doe",
Age: 45,
Email: "[email protected]"
},
{
Name: "Maria Smith",
Age: 23,
Email: "[email protected]"
},
{
Name: "Mike Miller",
Age: 33,
Email: "[email protected]"
}
);
How to view a PowerApps Collection? / How do I display a collection?
If your App gets complex it is getting hard to follow what is the content of your collections. Fortunately it is possible to check the current status of your variables and your collections.
Every collection that is existing within a Power App can be seen. To view the content of a collection, proceed as follows:
- Select “View” in the menu
- Select ”Collections” in the submenu
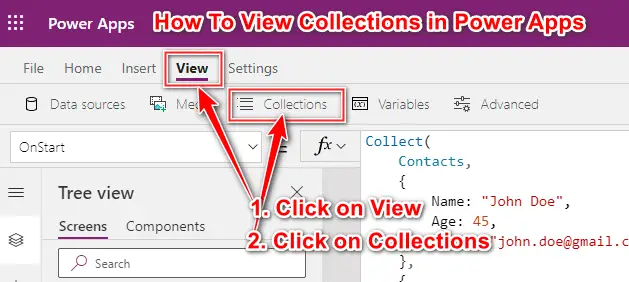
After clicking on “View” you will see all your collections and by clicking on them you will its content.
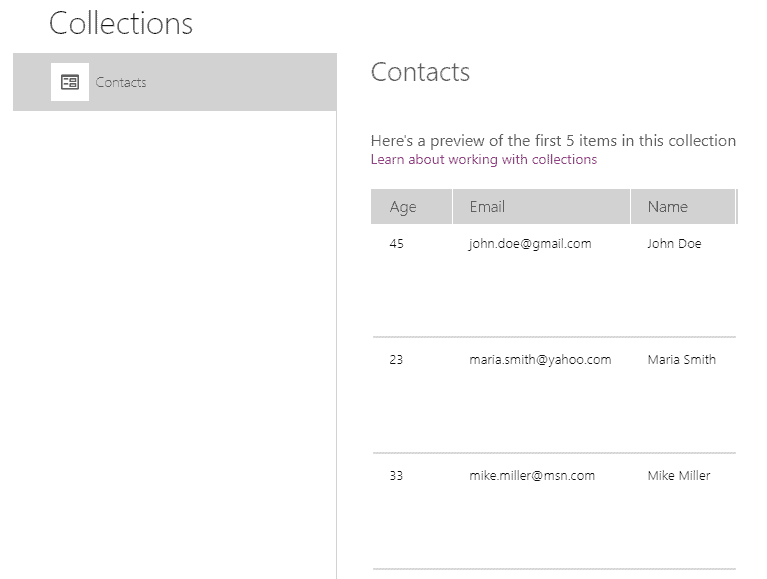
How do I delete a collection? / How do I clear a collection? / Remove multiple items
To remove all items in a PowerApps collection, use the Clear-function like this:
Clear(Contacts);
How to add an item
In contrast to ClearCollect Collect just adds the new record to the collection in PowerApps,
Collect(
Contacts,
{
Name: "Sally Smith",
Age: 67,
Email: "[email protected]"
}
);
How to sort a collection?
To sort a PowerApps collection use the Sort-Function. The Sort-Function has three types of parameters:
- Collection to be sorted
- Fields to be sorted by (can be multiple)
- Optional: Sort order (Ascending = default or Descending)
See the examples below. (The creation of the Contacts collection can be found here. )
Sort(["B","C","A"],Value);
// Returns A,B,C
Sort(["B","C","A"],Value,Descending);
// Returns C,B,A
Sort(Contacts,Age);
// Returns the Contacts sorted by Age (ascending)
Sort(Contacts,Age,Descending);
// Returns the Contacts sorted by Age (descending)
Sort(Contacts,Age,Email);
// Returns the Contacts sorted by Age and Email (ascending)
How to filter a collection?
To filter a PowerApps collection, you can use the Filter-Function. The Filter-Function needs to types of parameters:
- The collection that needs to be filtered
- The filter conditions (can be multiple)
Note: Multiple filter conditions are connected with an AND. In other words: all filter conditions must be true, not one or the other.
See the examples below. (The creation of the Contacts collection can be found here. )
ClearCollect(Numbers, [1,2,3,4]);
ClearCollect(Numbers,Filter(Numbers,Value>2));
// Sets the Numbers collection to to 3,4
ClearCollect(Numbers, [1,2,3,4]);
ClearCollect(Numbers,Filter(Numbers,Value>2,Value<4));
// Sets the Numbers collection to to 3,4
ClearCollect(ContactsAbove30,Filter(Contacts,Age>30));
// Set ContactsAbove30 to Contacts with Age greater than 30.