If statements are the bread and butter of programming environments. Within Microsoft Power Automate If statements can be formulated in two ways.
One option is to integrate a Power Automate Condition action as a step into a flow. This way you can open two separate branches within your flow based on the result of the condition.
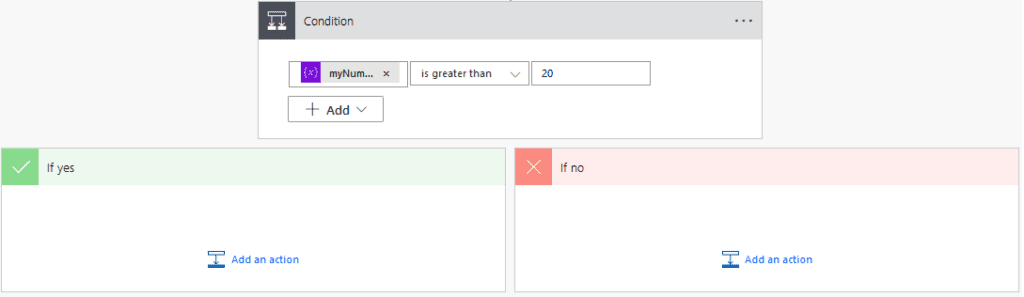
The other option is to use the Power Automate if function within an expression. This allows you to assign values based on a condition.
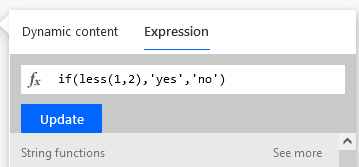
Although there are a lot of similarities between the Power Automate condition action and the if function, this article focus primarlly on the Power Automate if function.
The article is split into three major parts. Within these parts you will learn:
- Syntax of the Microsoft Power Automate If Function
- How to write if expression in Power Automate
- How to use if statements in common scenarios (lots of examples)
Power Automate If Function (Power Automate If Else)
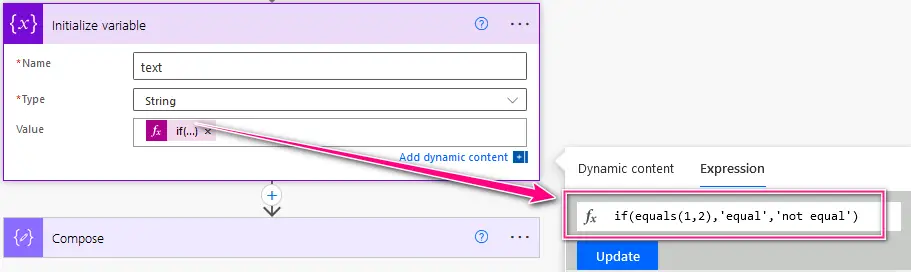
The If function lets you define conditional logic based on a boolean value. This boolean value is in most cases the result of Power Automate if condition expression that evaluates to a boolean.
Based on the boolean value, you can define a return value for the true and false path. It is basically a Power Automate If Else function.
There is no direct to do a if, else if, else statement. You need to nest if expressions to achieve if, else if, else,
Syntax
if(expression: boolean, valueIfTrue: any, valueIfFalse: any)
Parameters
- expression (mandatory): Boolean value (true or false). In most cases this will be an expression that evaluates to a boolean value.
- valueIfTrue (mandatory): Return value of the Power Automate If function if the expression is true.
- valueIfFalse (mandatory): Return value of the Power Automate If function if the expression is false.
Return value
- valueIfTrue, if Power Automate if statement condition is true.
- valueIfFalse, if Power Automate if statement condition is false.
Example
if(true,'yes','no')
=> "yes"
if(false,'yes','no')
=> "no"
if(equals(1,1),'equal','not equal')
=> "equal"
if(equals(1,2),'equal','not equal')
=> "not equal"
How to write if conditions in Power Automate
In most cases you will use a more or less complex expression in your if clause. So formulating the expression for your if condition is the hardest part.
The condition of your if statement need to evaluate to a boolean value. Power Automate provides a couple of functions that return a boolean value.
To understand what is your toolbox for your condition you need to take look at the functions that return a boolean value.
Here is an overview of all functions that are good candidates for your Power Automate If conditions.
bool
The bool function takes one argument and returns true if the argument is 1, true or a string ‘true’.
bool(1)
=> true
bool(0)
=> false
bool('true')
=> true
bool(true)
=> true
contains
The contains function takes two arguments and returns true if both values are true.
contains('hello','e')
=> true
contains(createArray(1,2,3),2)
=> true
empty
The empty function takes one argument and returns true if the argument is an empty string, null or an empty array.
empty('')
=> true
empty(' ')
=> false
empty(null)
=> true
Comparing values
endsWith
The endsWith function takes two string arguments and returns true if the first argument ends with she second one.
endsWith('end','nd')
=> true
equals
The equals function takes two arguments and returns true if both values are the same.
equals(1,1)
=> true
equals('abc','abc')
=> true
less
The less function takes two arguments and returns true if the first argument is less than the second. (Works for integer, float and string arguments)
less(1,2)
=> true
lessOrEquals
The lessOrEquals function takes two arguments and returns true if the first argument is less than the second or equals to the second argument. (Works for integer, float and string arguments)
lessOrEquals(1,2)
=> true
lessOrEquals(1,1)
=> true
greater
The greater function takes two arguments and returns true if the first argument is greater than the second. (Works for integer, float and string arguments)
greater(2,1)
=> true
greaterOrEquals
The greaterOrEquals function takes two arguments and returns true if the first argument is greater than the second or equals to the second argument. (Works for integer, float and string arguments)
greaterOrEquals(2,1)
=> true
greaterOrEquals(1,1)
=> true
startsWith
The startsWith function takes two string arguments and returns true if the first argument starts with she second one.
startsWith('start','st')
=> true
Combining Expressions
and
The and function takes two boolean arguments and returns true if both values are true.
and(less(18,10),greater(18,19))
=> true
or
The or function takes two boolean arguments and returns true if one value is true.
or(less(18,10),greater(18,19))
=> true
not
The not function takes one boolean argument and returns true if the argument is false.
not(startsWith('start','st'))
=> false
not(startsWith('start','xyz'))
=> true
if
Since the if function itself can return any type, you can also return boolean values. You can also nest if function this.
if(greater(1,2),false,true)
=> true
Power Automate If Expression Examples
Here are some common Power automate if statement examples. I hope they’ll help you.
Check if date field is empty
A classic Power Automate if condition example is a check if variable is empty.
This is the if expression to check for emptiness.
if(empty(variables('date')),'empty','not empty')
With a condition, you can do the same:
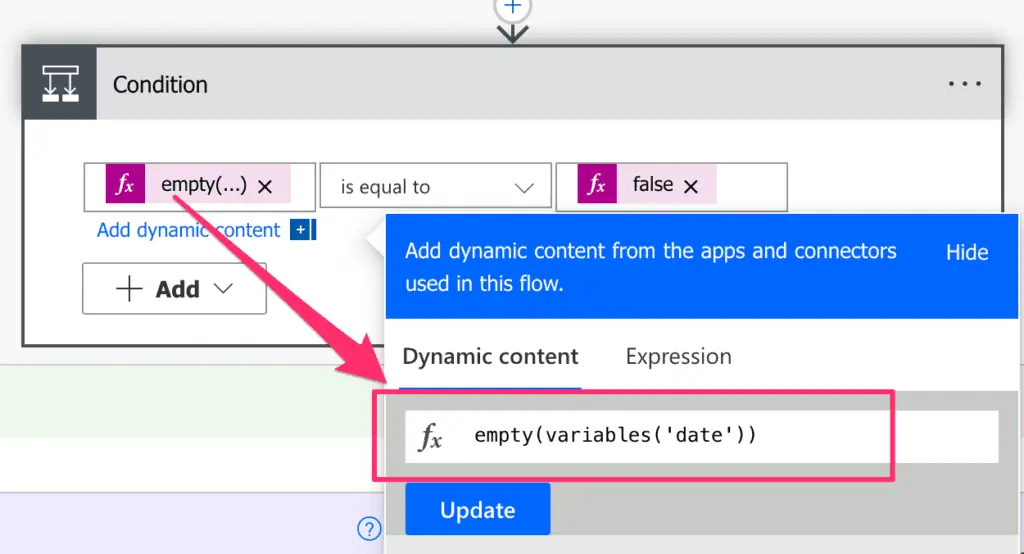
Check if array contains value
To check whether an element is in an array, combine contains and if:
if(contains(variables('myArray'),2),'yes','no')
With a condition you can do the same by doing this:
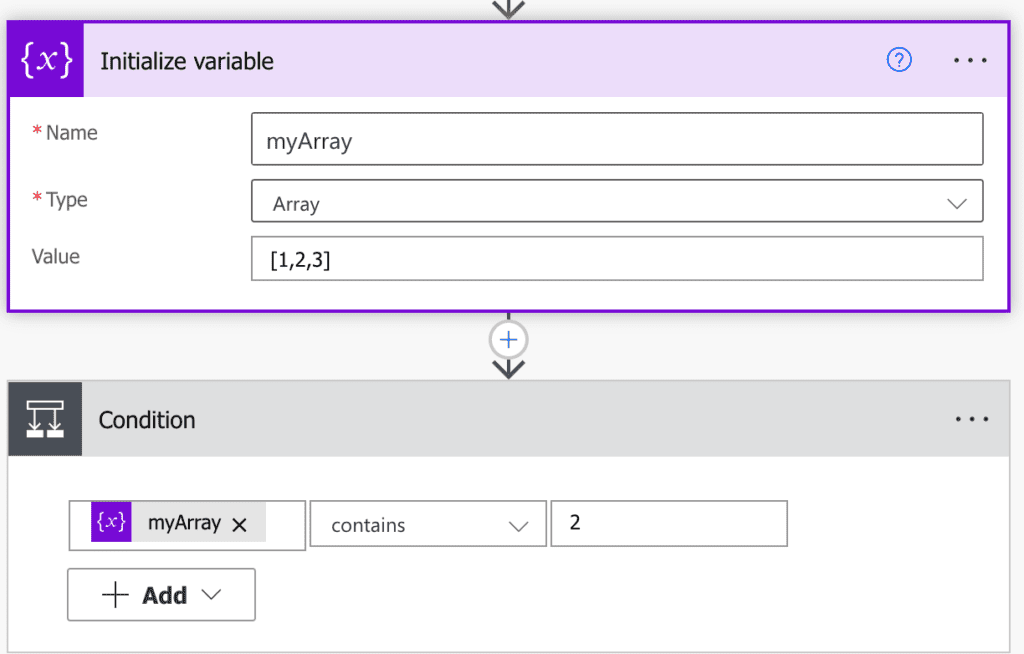
Check if array is empty
A classic Power Automate if condition example is a check if variable is empty.
This is the if expression to check for emptiness.
if(empty(variables('myArray')),'empty','not empty')
With a condition you can do the same by doing this:
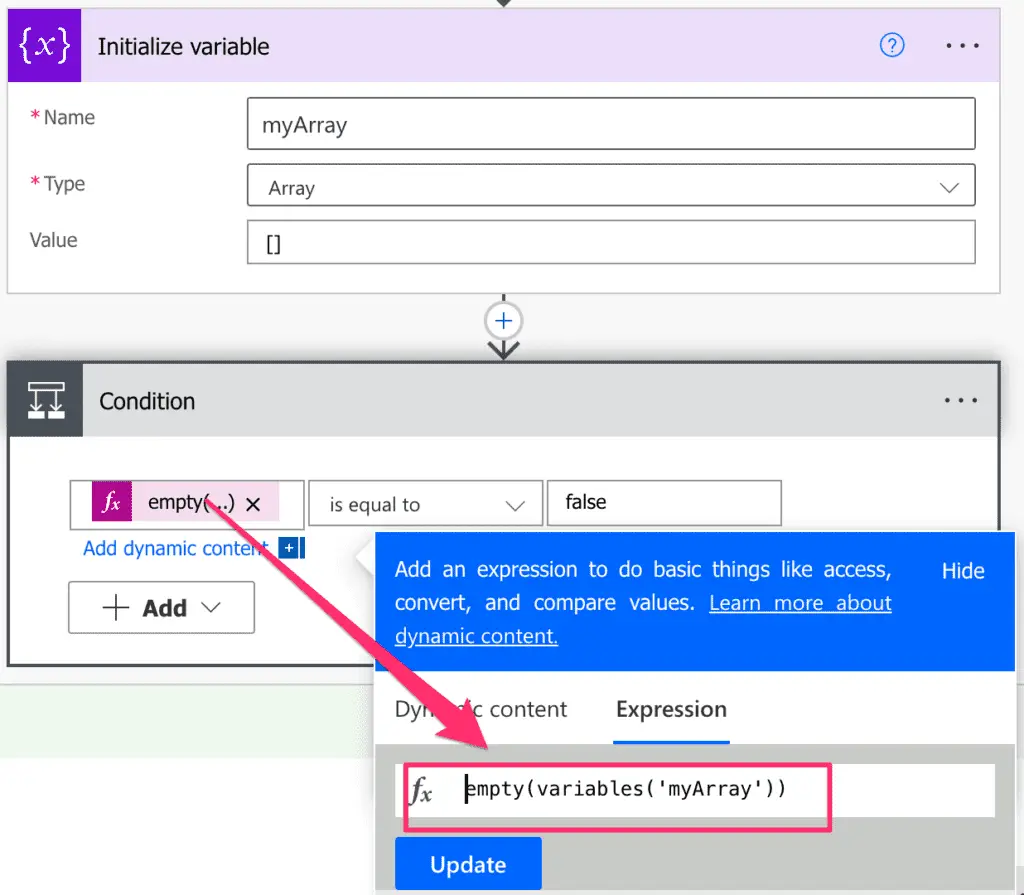